What is JS window.event, why it is deprecated and what to use instead?
November 12, 2021 ≈ 1 minute 46 seconds
A lot of times I used the event variable in my Javascript functions, saw «Deprecated symbol used, consult docs for better alternative» warning from my IDE, and just ignored it. Day-by-day, curiosity for what is event, why it is deprecated, and what should I use instead reached the level where I decided to learn about the subject.

Inside every Javascript function, called using HTML onevent attribute, event variable is accessible. So it must be associated with Window object. Let's check it:
<button onclick="clickMe()">Click me!</button>
<script>
function clickMe() {
console.log(window.event); // PointerEvent {...}
console.log(event === window.event)
}
</script>
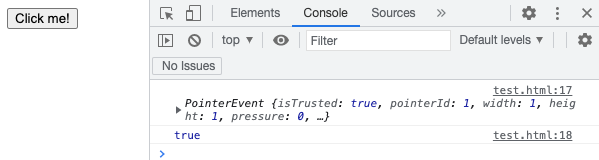
So, it's read-only window property, like MDN docs tells us: «The read-only Window property event returns the Event which is currently being handled by the site's code. Outside the context of an event handler, the value is always undefined.»
Also, that page has a big red deprecation warning, which tells us to avoid using it: «Avoid using it, and update existing code if possible»
So, what that IDE warning is all about?
— To stop using event, associated to Window object and use Event object passed to event listeners instead.
target.addEventListener(type, listener)
« It allows adding more than one handler for an event. This is particularly useful for libraries, JavaScript modules, or any other kind of code that needs to work well with other libraries or extensions.
In contrast to using an onXYZ property, it gives you finer-grained control of the phase when the listener is activated (capturing vs. bubbling).
It works on any event target, not just HTML or SVG elements. » — benefits, according to MDN
How the code above should look like?
<button>Click me!</button>
<script>
document.getElementsByTagName('button')[0].addEventListener('click', clickMe)
function clickMe(e) {
// e is the Event object, which passed as an argument to the event listener’s callback
console.log(e);
}
</script>
And what if I want clickMe to have some parameters? — Use anonymous function then:
<button>Click me!</button>
<script>
document.getElementsByTagName('button')[0].addEventListener('click', (ev) => clickMe(1, ev))
function clickMe(count, ev) {
// ev is the Event object, which passed as an argument to the event listener’s callback
console.log(count);
console.log(ev);
}
</script>
PS: Events section of WHATWG DOM Standard
You didn't provide any data, entered invalid email or already subscribed.
Try again. And if you still can't subscribe—write us.
Address successfully subscribed!
Subscribe to our newsletter
If you provide url of your website, we send you free design concept of one element (by our choice)
Subscribing to our newsletter, you comply with subscription terms and Privacy Policy